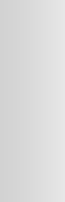 | | 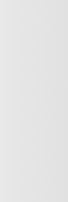 |

Its main design principles are ...
- Simplicity
- Expressiveness
- Safety
- Orthogonality
- Efficiency
First example: The famous program "hello world"
outl("hello world !")
Second example: User-defined control structures
# a) iterate over any objects
proc for(any :$ x; args a; code c) { a.for(x) c.exec() }
for(i,0..9) outl(i) # count from 0 to 9
# b) a loop similar to C, C++ or Java
proc Cfor(code c0,c1,c2,c3) { c0.exec() sys:for { if(! c1.eval()) break(1) c3.exec() c2.exec() } }
Cfor{ i = 0 }{ i <= 9 }{ i += 1 } { outl(i) } # count from 0 to 9
# c) a do-while loop
proc do(code b,c) { sys:for { b.exec() if(! c.eval()) break(1) } }
i = 0 do { outl(i); i += 1 } #while { i <= 9 } # count from 0 to 9
Features
- Control structures can be defined by the user (type code)
- Easy implementation of iterators (using types any and code)
- Application-specific extension of types
- Multiple inheritance of user-defined types
- Polymorphism for built-in and user-defined types (type any)
- Exception handling
- Strict separation between procedures and functions
- Overloading of procedures, functions, and operators
- Symbolic call of procedures and functions
- Variable number of arguments for procedures and functions (type args)
- Multiple number of function return values
- Variable number of function return values (type args)
- Multiple assignments within one statement
- Variable break of nested loops
- Generic read and write procedures for all types (pin, pout)
- Generic stream procedures for strings, files, and the console (in, out)
- No need to implement copy constructors (generic operator '=')
- No need to implement comparison operators (generic operator '==')
- No need to implement destructors (generic procedures clear and del)
- Dynamic data structures without new/delete operator (operator '~')
- Strong data typing, no implicit type conversion
- Strict data initialisation
- No free or dangling pointers
- No arithmetic based on pointers
- No memory leaks without using a garbage collector
- Several range operators
- Numeric data types with arbitrary length
- Several built-in container types (lists, sets, hash arrays, graphs, etc.)
- Iterators for container types, strings, files, and directories
- Types, procedures, and functions can be nested
- Comments can be nested
- No need for declarations
- No need for header files
- No need for makefiles (module inclusion)
- Almost every built-in type can be implemented with Nepal
History
28.08.2010 | | Nepal 1.0 released | 09.09.2011 | | Nepal 1.1 released | 05.01.2021 | | Nepal 1.2 released | 05.05.2024 | | Nepal 1.3 released |
Platforms
Windows, MacOS, Linux
License
Nepal is freeware.
Please refer to "About this site" for detailed terms of the license.
| 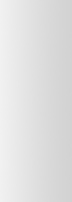 |